模擬
簡介
使用 Playwright,你可以在任何瀏覽器上測試你的應用程式,並模擬真實設備,例如手機或平板電腦。只需配置你想要模擬的設備,Playwright 就會模擬瀏覽器行為,例如 "userAgent"
、"screenSize"
、"viewport"
以及是否啟用 "hasTouch"
。你還可以模擬 "geolocation"
、"locale"
和 "timezone"
,對所有測試或特定測試進行設定,並設置 "permissions"
來顯示通知或更改 "colorScheme"
。
裝置
Playwright 附帶一個設備參數註冊表,使用 playwright.devices 為選定的桌面、平板和移動設備。它可以用來模擬特定設備的瀏覽器行為,例如使用者代理、螢幕大小、視窗大小以及是否啟用了觸控。所有測試將使用指定的設備參數進行。
- Test
- Library
import { defineConfig, devices } from '@playwright/test'; // import devices
export default defineConfig({
projects: [
{
name: 'chromium',
use: {
...devices['Desktop Chrome'],
},
},
{
name: 'Mobile Safari',
use: {
...devices['iPhone 13'],
},
},
],
});
const { chromium, devices } = require('playwright');
const browser = await chromium.launch();
const iphone13 = devices['iPhone 13'];
const context = await browser.newContext({
...iphone13,
});
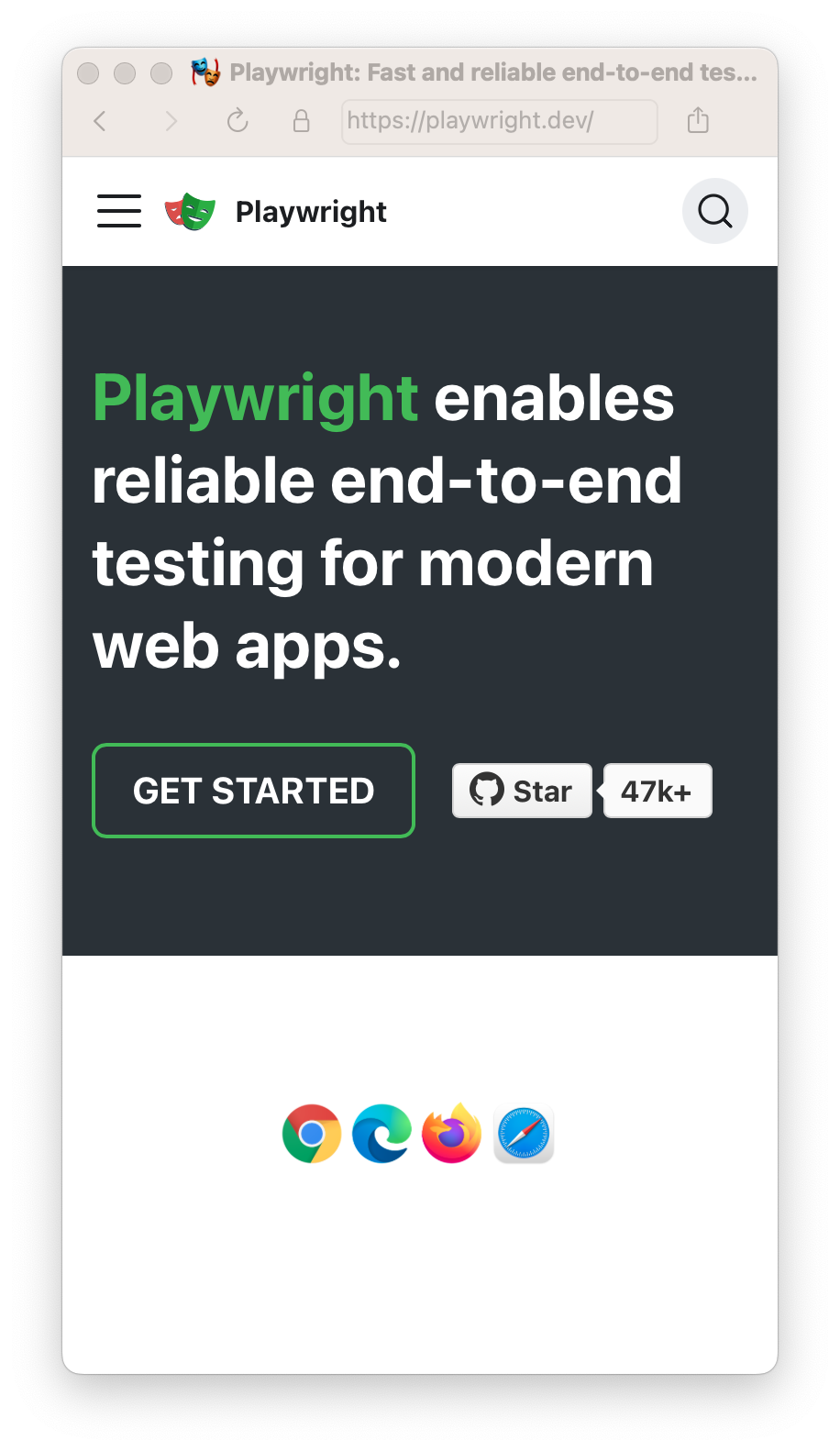
視口 (Viewport)
視口包含在裝置中,但您可以使用 page.setViewportSize() 覆蓋某些測試。
- Test
- Library
import { defineConfig, devices } from '@playwright/test';
export default defineConfig({
projects: [
{
name: 'chromium',
use: {
...devices['Desktop Chrome'],
// It is important to define the `viewport` property after destructuring `devices`,
// since devices also define the `viewport` for that device.
viewport: { width: 1280, height: 720 },
},
},
]
});
// Create context with given viewport
const context = await browser.newContext({
viewport: { width: 1280, height: 1024 }
});
測試檔案:
- Test
- Library
import { test, expect } from '@playwright/test';
test.use({
viewport: { width: 1600, height: 1200 },
});
test('my test', async ({ page }) => {
// ...
});
// Create context with given viewport
const context = await browser.newContext({
viewport: { width: 1280, height: 1024 }
});
// Resize viewport for individual page
await page.setViewportSize({ width: 1600, height: 1200 });
// Emulate high-DPI
const context = await browser.newContext({
viewport: { width: 2560, height: 1440 },
deviceScaleFactor: 2,
});
在測試文件內部也是一樣的。
- Test
- Library
import { test, expect } from '@playwright/test';
test.describe('specific viewport block', () => {
test.use({ viewport: { width: 1600, height: 1200 } });
test('my test', async ({ page }) => {
// ...
});
});
// Create context with given viewport
const context = await browser.newContext({
viewport: { width: 1600, height: 1200 }
});
const page = await context.newPage();
isMobile
無論是否考慮 meta viewport 標籤並啟用觸控事件。
import { defineConfig, devices } from '@playwright/test';
export default defineConfig({
projects: [
{
name: 'chromium',
use: {
...devices['Desktop Chrome'],
// It is important to define the `isMobile` property after destructuring `devices`,
// since devices also define the `isMobile` for that device.
isMobile: false,
},
},
]
});
Locale & Timezone
模擬使用者的區域和時區,可以在配置中全域設定所有測試,然後為特定測試覆蓋設定。
import { defineConfig } from '@playwright/test';
export default defineConfig({
use: {
// Emulates the user locale.
locale: 'en-GB',
// Emulates the user timezone.
timezoneId: 'Europe/Paris',
},
});
- Test
- Library
import { test, expect } from '@playwright/test';
test.use({
locale: 'de-DE',
timezoneId: 'Europe/Berlin',
});
test('my test for de lang in Berlin timezone', async ({ page }) => {
await page.goto('https://www.bing.com');
// ...
});
const context = await browser.newContext({
locale: 'de-DE',
timezoneId: 'Europe/Berlin',
});
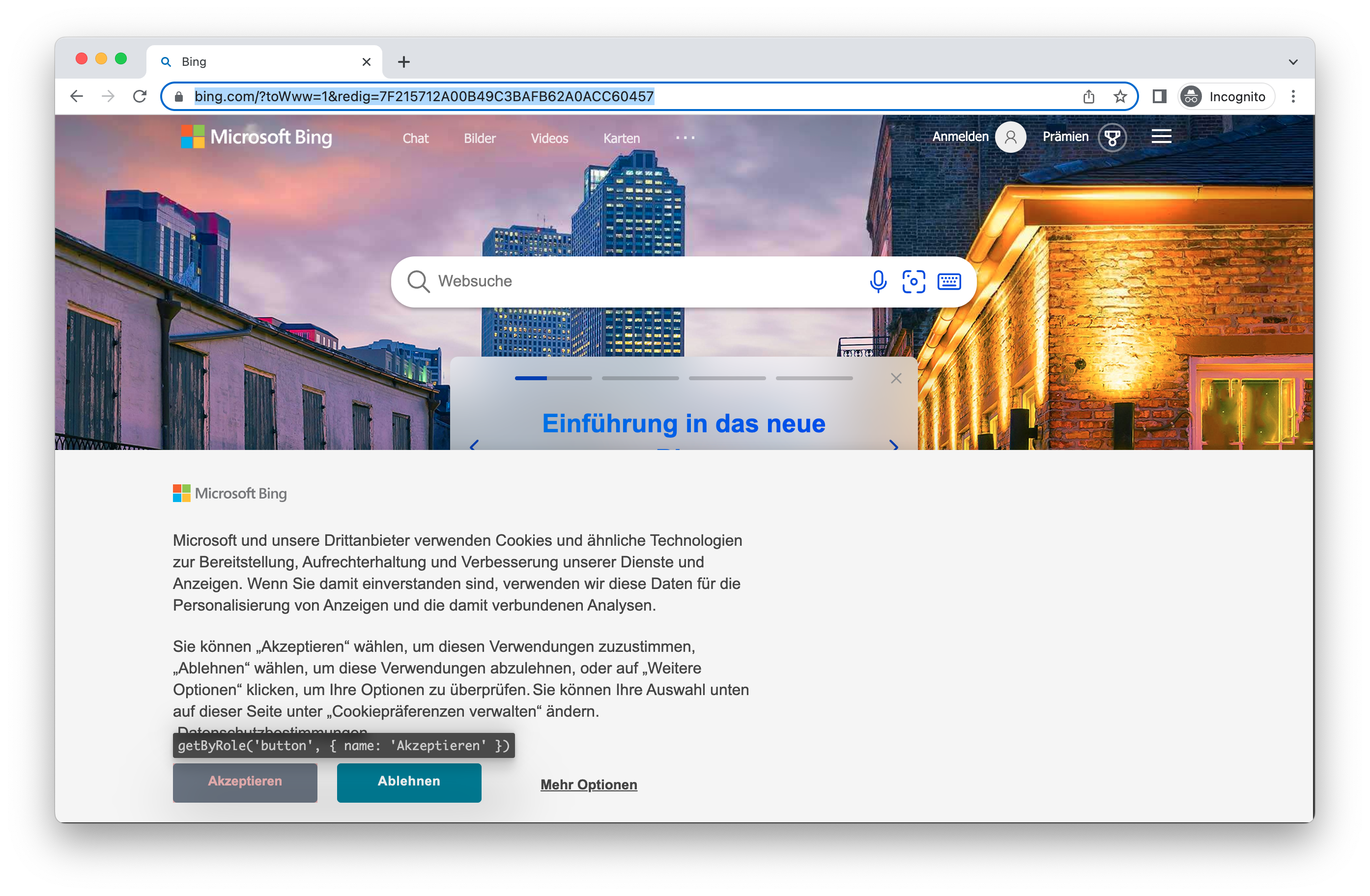
權限
允許應用程式顯示系統通知。
- Test
- Library
import { defineConfig } from '@playwright/test';
export default defineConfig({
use: {
// Grants specified permissions to the browser context.
permissions: ['notifications'],
},
});
const context = await browser.newContext({
permissions: ['notifications'],
});
允許特定域的通知。
- Test
- Library
import { test } from '@playwright/test';
test.beforeEach(async ({ context }) => {
// Runs before each test and signs in each page.
await context.grantPermissions(['notifications'], { origin: 'https://skype.com' });
});
test('first', async ({ page }) => {
// page has notifications permission for https://skype.com.
});
await context.grantPermissions(['notifications'], { origin: 'https://skype.com' });
撤銷所有權限,請使用 browserContext.clearPermissions()。
// Library
await context.clearPermissions();
地理位置
授予 "geolocation" 權限並將 geolocation 設定到特定區域。
import { defineConfig } from '@playwright/test';
export default defineConfig({
use: {
// Context geolocation
geolocation: { longitude: 12.492507, latitude: 41.889938 },
permissions: ['geolocation'],
},
});
- Test
- Library
import { test, expect } from '@playwright/test';
test.use({
geolocation: { longitude: 41.890221, latitude: 12.492348 },
permissions: ['geolocation'],
});
test('my test with geolocation', async ({ page }) => {
// ...
});
const context = await browser.newContext({
geolocation: { longitude: 41.890221, latitude: 12.492348 },
permissions: ['geolocation']
});
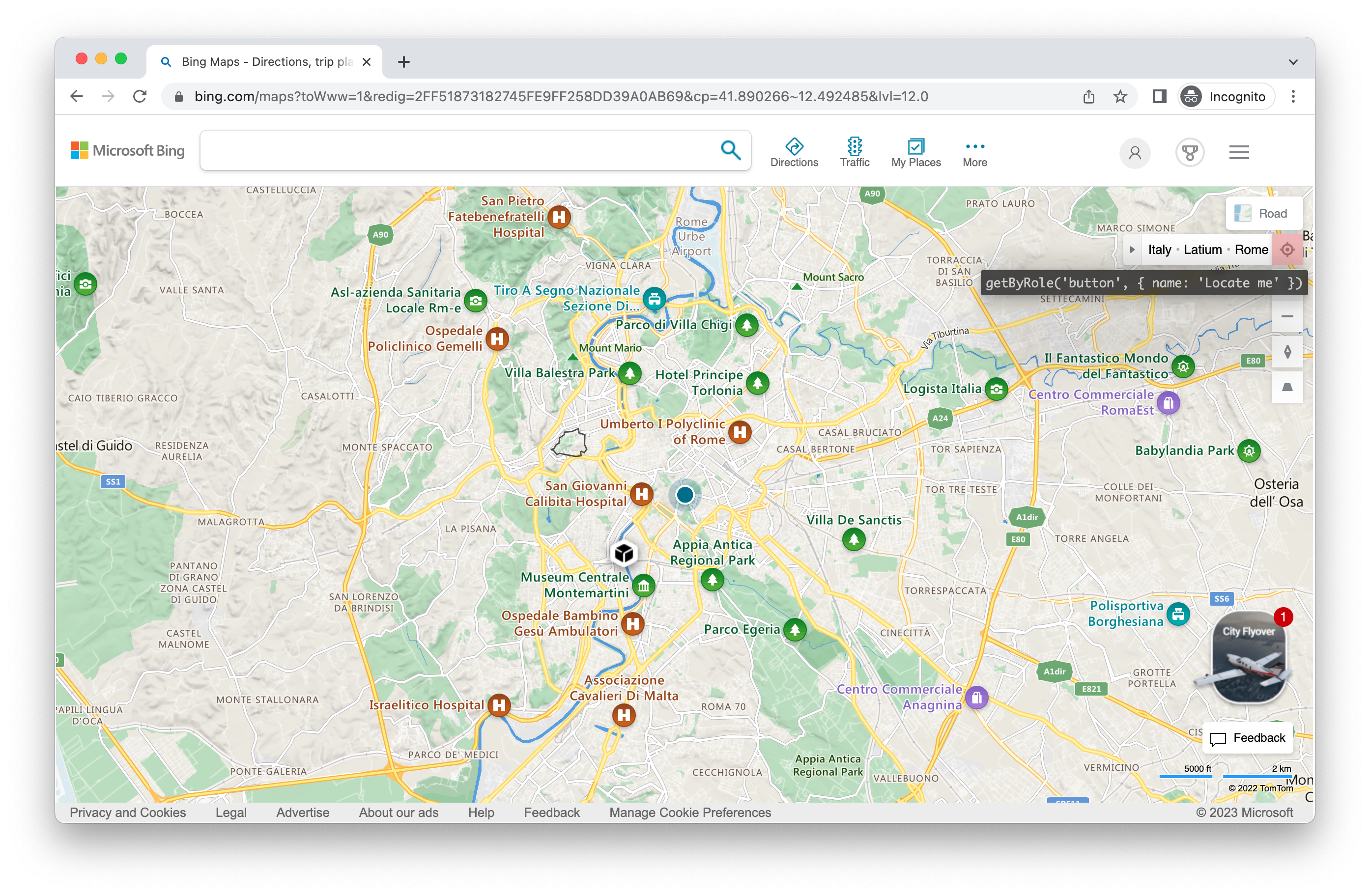
稍後更改位置:
- Test
- Library
import { test, expect } from '@playwright/test';
test.use({
geolocation: { longitude: 41.890221, latitude: 12.492348 },
permissions: ['geolocation'],
});
test('my test with geolocation', async ({ page, context }) => {
// overwrite the location for this test
await context.setGeolocation({ longitude: 48.858455, latitude: 2.294474 });
});
await context.setGeolocation({ longitude: 48.858455, latitude: 2.294474 });
注意 您只能更改所有頁面的地理位置。
色彩方案和媒體
模擬使用者的"colorScheme"
。支援的值有 'light'、'dark'、'no-preference'。你也可以使用 page.emulateMedia() 模擬媒體類型。
import { defineConfig } from '@playwright/test';
export default defineConfig({
use: {
colorScheme: 'dark',
},
});
- Test
- Library
import { test, expect } from '@playwright/test';
test.use({
colorScheme: 'dark' // or 'light'
});
test('my test with dark mode', async ({ page }) => {
// ...
});
// Create context with dark mode
const context = await browser.newContext({
colorScheme: 'dark' // or 'light'
});
// Create page with dark mode
const page = await browser.newPage({
colorScheme: 'dark' // or 'light'
});
// Change color scheme for the page
await page.emulateMedia({ colorScheme: 'dark' });
// Change media for page
await page.emulateMedia({ media: 'print' });
使用者代理
使用者代理包含在裝置中,因此您很少需要更改它。但是,如果您需要測試不同的使用者代理,您可以使用 userAgent
屬性來覆寫它。
- Test
- Library
import { test, expect } from '@playwright/test';
test.use({ userAgent: 'My user agent' });
test('my user agent test', async ({ page }) => {
// ...
});
const context = await browser.newContext({
userAgent: 'My user agent'
});
離線
模擬網路離線。
import { defineConfig } from '@playwright/test';
export default defineConfig({
use: {
offline: true
},
});
啟用 JavaScript
模擬使用者在 JavaScript 被停用的情境。
- Test
- Library
import { test, expect } from '@playwright/test';
test.use({ javaScriptEnabled: false });
test('test with no JavaScript', async ({ page }) => {
// ...
});
const context = await browser.newContext({
javaScriptEnabled: false
});